- Authors
- Name
- The Alchemist
- @dnwemedia
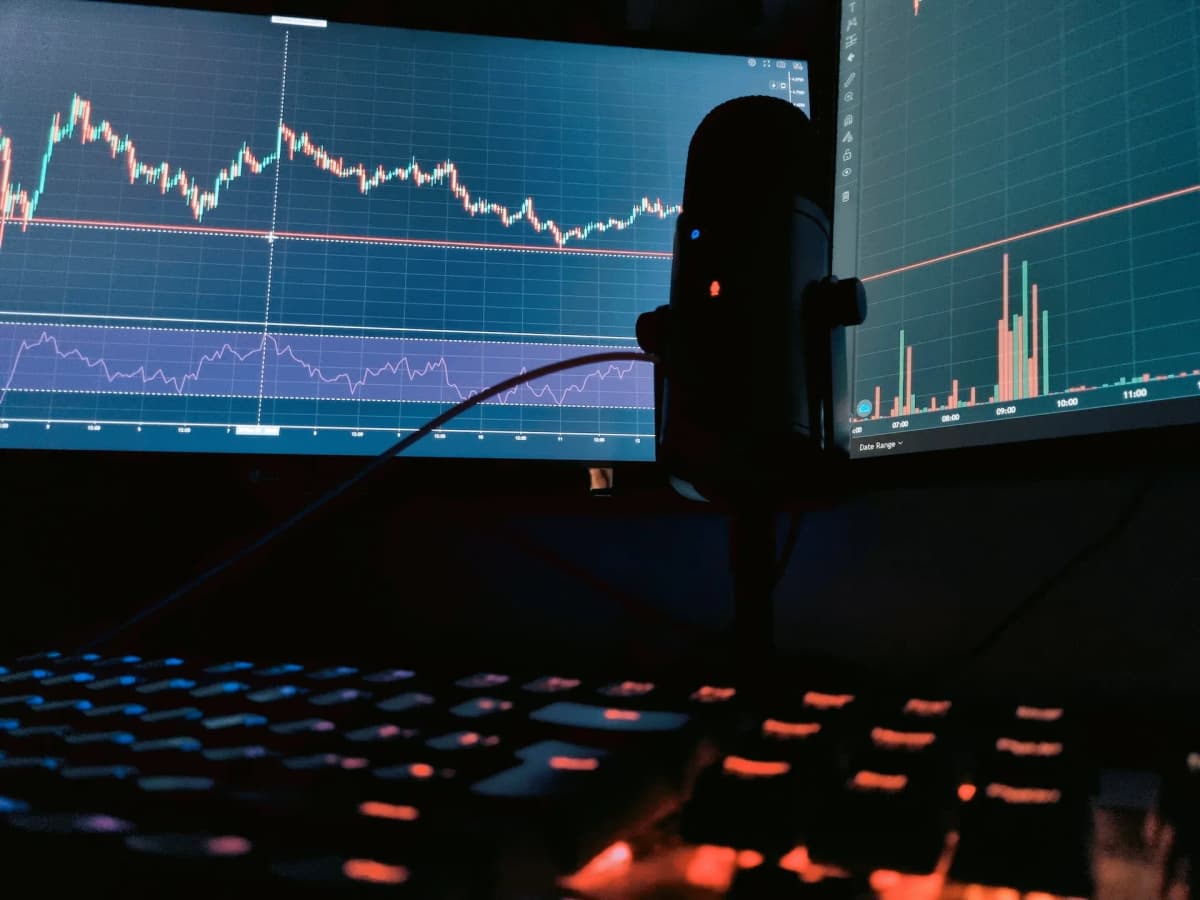
Solana program development using Rust offers unparalleled speed and efficiency. This article explores essential concepts, best practices, and optimization techniques for building robust Solana programs.
Table of Contents
- Introduction to Solana Program Development with Rust
- Understanding Solana's Architecture
- Setting Up Your Development Environment
- Core Concepts in Solana Program Development
- Writing Your First Solana Program in Rust
- Best Practices for Solana Program Development
- Advanced Topics in Solana Program Development
- Optimizing Solana Programs for Performance
- Security Considerations
- Conclusion
- Frequently Asked Questions
Introduction to Solana Program Development with Rust
Solana has emerged as a leading blockchain platform renowned for its high throughput and low transaction costs. Unlike many other blockchains, Solana achieves its remarkable performance through a unique architecture and a programming model optimized for speed. Rust, a systems programming language known for its safety and performance, is the primary language for developing Solana programs (also known as smart contracts). This article delves into the core concepts of Solana program development with Rust, providing developers with the knowledge and best practices needed to build efficient and secure decentralized applications.
Understanding Solana's Architecture
Solana's architecture differs significantly from traditional blockchains like Ethereum. It employs a Proof-of-History (PoH) consensus mechanism combined with a Proof-of-Stake (PoS) mechanism, enabling parallel transaction processing and significantly reducing block times. Key components of Solana's architecture include:
- Sealevel: A parallel transaction processing engine that allows multiple transactions to be executed concurrently, maximizing throughput.
- Tower BFT: Solana's implementation of Practical Byzantine Fault Tolerance (pBFT), optimized for high performance.
- Gulf Stream: A transaction forwarding protocol that reduces confirmation times by predicting future validators.
- Turbine: A block propagation protocol that enables efficient distribution of data across the network.
Understanding these architectural components is crucial for writing Solana programs that can take full advantage of the platform's capabilities.
Setting Up Your Development Environment
Before diving into coding, it's essential to set up a suitable development environment. This typically involves installing the Solana tool suite, Rust, and other necessary dependencies.
- Install Rust: If you don't have Rust installed, you can download it from the official Rust website (https://www.rust-lang.org/). Use
rustup
to manage Rust versions and components. - Install Solana Tool Suite: The Solana tool suite includes the Solana CLI, which is essential for deploying and interacting with Solana programs. You can install it by following the instructions on the Solana documentation (https://docs.solana.com/cli/install).
- Configure Your Shell: Add the Solana CLI to your PATH environment variable to make it accessible from your terminal.
- Install Anchor (Optional): Anchor is a framework that simplifies Solana program development by providing higher-level abstractions and tools. While not required, it can significantly streamline the development process. You can install it using
cargo install anchor-cli
.
Core Concepts in Solana Program Development
Solana program development revolves around several key concepts:
- Accounts: Accounts are the fundamental data storage units in Solana. They hold data, lamports (Solana's native token), and metadata. Programs interact with accounts to read and modify their data.
- Programs: Programs are executable code deployed on the Solana blockchain. They define the logic and rules for interacting with accounts.
- Instructions: Instructions are the actions that programs perform. They specify the program to be executed, the accounts to be accessed, and any input data.
- Transactions: Transactions are bundles of instructions that are atomically executed on the Solana blockchain. They ensure that either all instructions succeed or none do.
- The Solana Program Model: Programs are stateless. All state is stored in accounts, and programs manipulate accounts based on instructions.
Writing Your First Solana Program in Rust
Let's walk through a simple example to illustrate the basics of Solana program development. We'll create a program that initializes an account and stores a simple message in it.
use solana_program::{
account_info::{next_account_info, AccountInfo},
entrypoint,
entrypoint::ProgramResult,
msg,
pubkey::Pubkey,
};
entrypoint!(process_instruction);
pub fn process_instruction(
program_id: &Pubkey,
accounts: &[AccountInfo],
instruction_data: &[u8],
) -> ProgramResult {
msg!("Hello, Solana!");
// Get the account iterator
let accounts_iter = &mut accounts.iter();
// Get the account to say hello to
let account = next_account_info(accounts_iter)?;
// The account must be owned by the program
if account.owner != program_id {
msg!("Account does not have the correct program id");
return Err(ProgramError::IncorrectProgramId);
}
// Increment and store the number of times the account has been greeted
let mut greeting_account = GreetingAccount::try_from_slice(&account.data.borrow())?;
greeting_account.counter += 1;
greeting_account.serialize(&mut &mut account.data.borrow_mut()[..])?;
msg!("Greeted {} time(s)!", greeting_account.counter);
Ok(())
}
This code defines a simple Solana program that logs a message and increments a counter in an account. Key parts of this code include:
entrypoint!
: This macro defines the entry point for the program.process_instruction
: This function is the main logic of the program. It takes the program ID, accounts, and instruction data as input.accounts
: An array ofAccountInfo
structs, which provide access to the accounts involved in the transaction.instruction_data
: The data associated with the instruction, which can be used to pass parameters to the program.
Best Practices for Solana Program Development
To write efficient and secure Solana programs, follow these best practices:
- Minimize On-Chain Data: Storing large amounts of data on-chain can be expensive and slow. Only store essential data on-chain and consider off-chain storage solutions for less critical information.
- Use Efficient Data Structures: Choose data structures that optimize for read and write operations. Consider using custom data structures tailored to your program's specific needs.
- Validate Inputs: Always validate inputs to prevent malicious or unexpected behavior. Ensure that accounts are owned by the correct programs and that instruction data is within expected bounds.
- Handle Errors Gracefully: Implement robust error handling to prevent programs from crashing or behaving unpredictably. Use the
Result
type to propagate errors and provide informative error messages. - Write Unit Tests: Thoroughly test your programs to ensure they function correctly and handle edge cases. Use the Solana test framework to write unit tests and integration tests.
- Profile and Optimize: Use profiling tools to identify performance bottlenecks and optimize your code accordingly. Pay attention to the number of compute units consumed by your program, as this directly affects transaction costs.
- Secure Your Program: Implement security best practices to protect against common vulnerabilities, such as integer overflows, underflows, and reentrancy attacks.
Advanced Topics in Solana Program Development
Once you've mastered the basics, you can explore more advanced topics in Solana program development:
- Cross-Program Invocation (CPI): CPI allows programs to invoke other programs, enabling complex interactions and composability.
- Program Derived Addresses (PDAs): PDAs are addresses that are derived from a program ID and a set of seeds. They provide a way for programs to create and manage accounts without requiring private keys.
- Upgradeable Programs: Solana supports upgradeable programs, allowing you to update your program logic without redeploying the entire program.
- State Compression: Techniques for reducing the amount of storage space required for on-chain data, such as using sparse Merkle trees.
Optimizing Solana Programs for Performance
Optimizing Solana programs for performance is crucial for minimizing transaction costs and maximizing throughput. Here are some optimization techniques:
- Reduce Compute Unit Consumption: Minimize the number of compute units consumed by your program by optimizing your code and reducing unnecessary operations.
- Batch Operations: Batch multiple operations into a single transaction to reduce transaction overhead.
- Use Efficient Algorithms: Choose algorithms that are optimized for performance and minimize computational complexity.
- Cache Data: Cache frequently accessed data to reduce the number of read operations from accounts.
- Avoid Loops: Minimize the use of loops, as they can be computationally expensive. Consider using alternative approaches, such as vectorized operations.
- Use the
#[inline]
Attribute: Use the#[inline]
attribute to encourage the compiler to inline frequently called functions, reducing function call overhead.
Security Considerations
Security is paramount in Solana program development. Here are some security considerations to keep in mind:
- Prevent Integer Overflows and Underflows: Use the
checked_add
,checked_sub
,checked_mul
, andchecked_div
methods to prevent integer overflows and underflows. - Guard Against Reentrancy Attacks: Reentrancy attacks occur when a program calls another program, which then calls back into the original program. Use techniques such as checks-effects-interactions to prevent reentrancy attacks.
- Sanitize Inputs: Sanitize all inputs to prevent injection attacks and other vulnerabilities.
- Use Audited Libraries: Use well-audited libraries and frameworks to reduce the risk of introducing vulnerabilities into your code.
- Follow Security Best Practices: Follow security best practices for Rust and Solana development to minimize the risk of vulnerabilities.
Conclusion
Solana program development with Rust offers a powerful platform for building high-performance decentralized applications. By understanding the core concepts, following best practices, and optimizing your code for performance and security, you can create robust and efficient Solana programs that push the boundaries of blockchain technology. As the Solana ecosystem continues to evolve, staying up-to-date with the latest developments and best practices is essential for success.
Frequently Asked Questions
Q1: What is the primary advantage of using Rust for Solana program development?
Rust offers memory safety and performance benefits, crucial for Solana's high-throughput environment. Its strict compiler helps prevent common programming errors.
Q2: How does Solana achieve high transaction speeds compared to other blockchains?
Solana uses Proof of History (PoH) combined with Proof of Stake (PoS), enabling parallel transaction processing and reduced block times.
Q3: What are accounts in Solana, and how are they used?
Accounts are fundamental data storage units in Solana, holding data, lamports, and metadata. Programs interact with accounts to read and modify their data.
Q4: What is Cross-Program Invocation (CPI) in Solana?
CPI allows programs to invoke other programs, enabling complex interactions and composability within the Solana ecosystem.
Q5: How can I optimize my Solana program for better performance?
Optimize by reducing compute unit consumption, batching operations, using efficient algorithms, caching data, and avoiding loops.