- Authors
- Name
- The Alchemist
- @dnwemedia
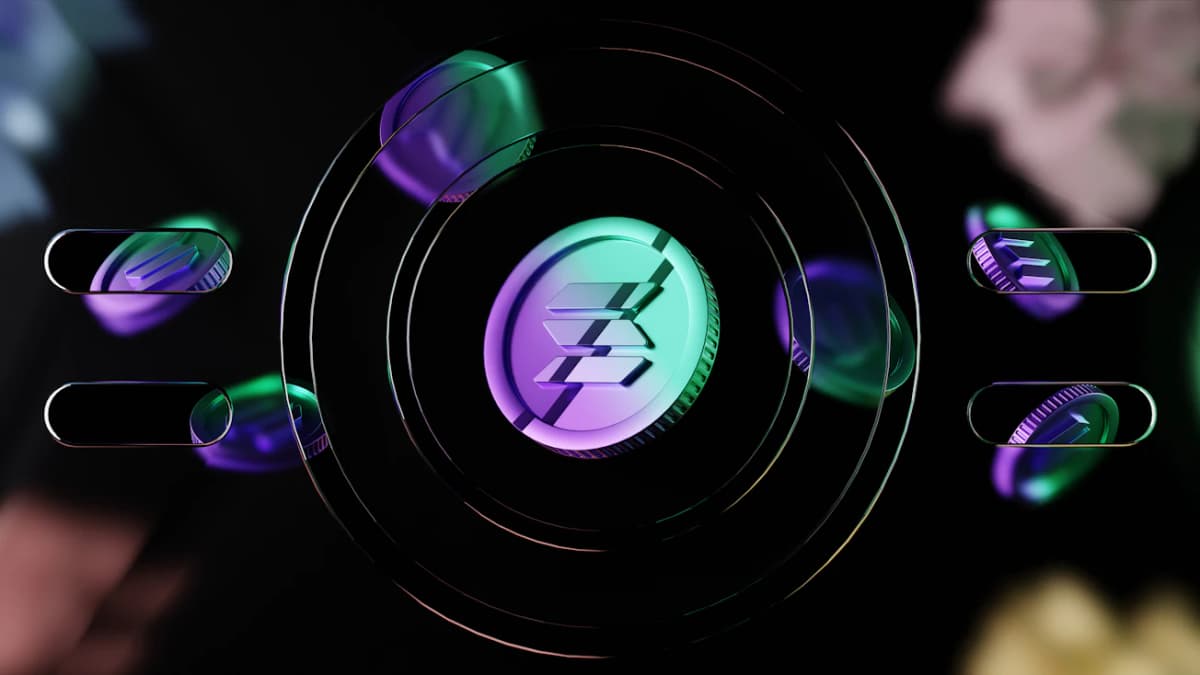
Unlock the power of cross-chain interoperability by connecting Solana and Ethereum using Wormhole in Rust. Explore the architecture, implementation, and potential of this innovative technology...
Table of Contents
- Introduction: The Need for Cross-Chain Interoperability
- Understanding Solana and Ethereum: A Comparative Overview
- Introducing Wormhole: A Decentralized Cross-Chain Messaging Protocol
- Setting Up Your Development Environment for Rust and Solana
- Writing a Solana Program to Interact with Wormhole
- Creating an Ethereum Contract to Receive Messages from Wormhole
- Bridging the Gap: Sending Messages from Solana to Ethereum
- Use Cases and Applications of Solana-Ethereum Interoperability
- Challenges and Considerations
- Conclusion: The Future of Interconnected Blockchains
- Frequently Asked Questions
Introduction: The Need for Cross-Chain Interoperability
In the ever-evolving landscape of blockchain technology, the existence of isolated ecosystems presents a significant challenge. Blockchains like Solana and Ethereum, while offering unique strengths and functionalities, operate independently, hindering seamless communication and asset transfer between them. This siloed nature limits the potential for collaboration and innovation within the broader blockchain space. Cross-chain interoperability emerges as a crucial solution, aiming to bridge these gaps and unlock a more interconnected and efficient blockchain future.
Imagine a world where digital assets can effortlessly flow between different blockchain networks, where decentralized applications (dApps) on one chain can seamlessly interact with smart contracts on another, and where developers can leverage the unique features of multiple chains to create innovative solutions. This is the promise of cross-chain interoperability, and it's becoming increasingly vital for the growth and mainstream adoption of blockchain technology.
Understanding Solana and Ethereum: A Comparative Overview
Before delving into the specifics of connecting Solana and Ethereum with Wormhole, it's essential to understand the key characteristics of each platform.
Solana:
- High Performance: Solana is renowned for its exceptional speed and scalability, achieving significantly higher transaction throughput than many other blockchains. This is primarily due to its innovative Proof of History (PoH) consensus mechanism, which enables parallel transaction processing.
- Low Transaction Fees: Solana's high efficiency translates to remarkably low transaction fees, making it an attractive platform for applications requiring frequent transactions, such as decentralized exchanges (DEXs) and micro-payments.
- Rust Programming Language: Solana's native programming language is Rust, a systems programming language known for its safety, performance, and memory management capabilities. This allows developers to build robust and secure smart contracts on the Solana blockchain.
Ethereum:
- Ecosystem Maturity: Ethereum boasts the largest and most mature ecosystem in the blockchain space, with a vast array of dApps, DeFi protocols, and developer tools.
- Smart Contract Functionality: Ethereum pioneered the concept of smart contracts, enabling developers to create complex and automated agreements that execute automatically when predefined conditions are met.
- Solidity Programming Language: Ethereum's primary programming language is Solidity, a high-level language specifically designed for writing smart contracts.
While both Solana and Ethereum offer powerful capabilities, their distinct architectures and programming languages create barriers to direct communication. This is where Wormhole steps in to provide a bridge between these two ecosystems.
Introducing Wormhole: A Decentralized Cross-Chain Messaging Protocol
Wormhole is a generic cross-chain messaging protocol that enables communication between different blockchain networks. It acts as a decentralized bridge, allowing data and assets to be transferred securely and efficiently between supported chains. Wormhole achieves this through a network of Guardians who observe and attest to events on connected chains.
Key Features of Wormhole:
- Decentralized: Wormhole operates as a decentralized network, eliminating the need for a central intermediary and reducing the risk of censorship or single points of failure.
- Generic Messaging: Wormhole supports the transfer of arbitrary data between chains, enabling a wide range of cross-chain applications beyond simple asset transfers.
- Security: Wormhole employs a robust security model, relying on a network of trusted Guardians to verify and attest to cross-chain messages.
- Efficiency: Wormhole is designed for speed and efficiency, enabling near-instantaneous cross-chain communication.
Wormhole's architecture allows it to connect a variety of blockchains, including Solana, Ethereum, Binance Smart Chain, and others. This makes it a versatile solution for building cross-chain applications and unlocking the potential of a multi-chain future.
Setting Up Your Development Environment for Rust and Solana
To begin building cross-chain applications with Wormhole on Solana, you'll need to set up your development environment. This involves installing the necessary tools and libraries, including Rust, Solana CLI, and Anchor.
Step 1: Install Rust:
If you don't already have Rust installed, you can download and install it from the official Rust website (https://www.rust-lang.org/). Follow the instructions for your operating system.
Step 2: Install Solana CLI:
The Solana CLI (Command-Line Interface) is essential for interacting with the Solana blockchain. You can install it by running the following command in your terminal:
sh -c "$(curl -sSfL https://release.solana.com/v1.10.31/install)"
Make sure to add the Solana CLI to your PATH environment variable.
Step 3: Install Anchor:
Anchor is a framework for building secure and efficient Solana programs (smart contracts). You can install it using the following command:
cargo install --git https://github.com/coral-xyz/anchor anchor-cli --locked
Step 4: Install Dependencies:
You'll also need to install some additional dependencies, such as npm
and yarn
:
sudo apt update
sudo apt install npm
npm install -g yarn
Once you have these tools installed, you're ready to start developing Solana programs with Rust and Anchor.
Writing a Solana Program to Interact with Wormhole
Now, let's dive into the code and write a Solana program that interacts with Wormhole. This program will demonstrate how to send a message from Solana to Ethereum using the Wormhole protocol.
Step 1: Create a New Anchor Project:
Create a new Anchor project using the following command:
anchor init wormhole_example
cd wormhole_example
Step 2: Define the Program Logic:
Open the lib.rs
file in the programs/wormhole_example
directory and add the following code:
use anchor_lang::prelude::*;
use wormhole_sdk::wormhole;
declare_id!("Fg6PaFpoGXkYsidMpWTk6W2BeZ7FEfcYkg476zPFsLn");
#[program]
pub mod wormhole_example {
use super::*;
pub fn send_message(ctx: Context<SendMessage>, message: String) -> Result<()> {
let wormhole_program_id = wormhole::program::ID;
// Construct the Wormhole message
let payload = message.as_bytes();
// Send the message to Wormhole
wormhole::send_message(
ctx.accounts.wormhole_config.to_account_info(),
ctx.accounts.message.to_account_info(),
ctx.accounts.payer.to_account_info(),
wormhole_program_id,
payload,
)?;
Ok(())
}
}
#[derive(Accounts)]
pub struct SendMessage<'info> {
#[account(mut)]
pub payer: Signer<'info>,
/// CHECK: Wormhole config account
pub wormhole_config: AccountInfo<'info>,
/// CHECK: Message account
#[account(mut)]
pub message: AccountInfo<'info>,
pub system_program: Program<'info, System>,
}
This code defines a simple Solana program with a single instruction, send_message
. This instruction takes a message as input and sends it to Wormhole using the wormhole::send_message
function.
Step 3: Define the Accounts: The SendMessage
struct defines the accounts required by the send_message
instruction. These accounts include the payer, the Wormhole config account, the message account, and the system program.
Step 4: Build and Deploy the Program:
Build the program using the following command:
anchor build
Deploy the program to the Solana blockchain using the following command:
anchor deploy
Creating an Ethereum Contract to Receive Messages from Wormhole
On the Ethereum side, you'll need to create a smart contract that can receive messages from Wormhole. This contract will listen for events emitted by the Wormhole relay and process the messages accordingly.
Step 1: Set Up Your Ethereum Development Environment:
You'll need to install Node.js and npm (Node Package Manager) if you don't already have them. Then, install Hardhat, a popular Ethereum development environment:
npm install -g hardhat
Create a new Hardhat project:
mkdir ethereum_wormhole
cd ethereum_wormhole
hardhat
Step 2: Define the Ethereum Contract:
Create a new Solidity file, WormholeReceiver.sol
, in the contracts
directory and add the following code:
pragma solidity ^0.8.0;
contract WormholeReceiver {
event MessageReceived(address sender, string message);
function receiveMessage(address _sender, string memory _message) public {
emit MessageReceived(_sender, _message);
}
}
This contract defines a single function, receiveMessage
, which takes the sender's address and the message as input. It then emits a MessageReceived
event, which can be listened to by other applications.
Step 3: Deploy the Ethereum Contract:
Deploy the contract to a local Ethereum network or a testnet using Hardhat. You'll need to configure your Hardhat environment and write a deployment script.
Bridging the Gap: Sending Messages from Solana to Ethereum
With the Solana program and the Ethereum contract in place, you can now send messages from Solana to Ethereum using Wormhole. This involves the following steps:
- Send a Message from Solana: Call the
send_message
instruction in your Solana program, providing the message you want to send to Ethereum. - Wormhole Observes the Message: The Wormhole Guardians observe the Solana blockchain and detect the message sent by your program.
- Guardians Attest to the Message: The Guardians collectively attest to the validity of the message and generate a verifiable attestation.
- Relay the Message to Ethereum: A relay service submits the attestation to the Wormhole contract on Ethereum.
- Ethereum Contract Receives the Message: The Wormhole contract on Ethereum verifies the attestation and calls the
receiveMessage
function in yourWormholeReceiver
contract, passing the sender's address and the message as input. - Process the Message: Your
WormholeReceiver
contract emits aMessageReceived
event, which can be listened to by other applications or processed further.
This process demonstrates how Wormhole enables secure and decentralized communication between Solana and Ethereum, allowing you to build cross-chain applications that leverage the strengths of both platforms.
Use Cases and Applications of Solana-Ethereum Interoperability
The ability to connect Solana and Ethereum opens up a wide range of exciting use cases and applications, including:
- Cross-Chain Asset Transfers: Seamlessly transfer tokens and other digital assets between Solana and Ethereum, enabling users to take advantage of the speed and low fees of Solana while still participating in the Ethereum ecosystem.
- Cross-Chain DeFi Protocols: Build DeFi protocols that operate across both Solana and Ethereum, allowing users to access a wider range of financial services and opportunities.
- Cross-Chain Gaming: Create gaming experiences that leverage the high performance of Solana for gameplay and the established ecosystem of Ethereum for asset management and ownership.
- Data Sharing and Oracles: Share data between Solana and Ethereum, enabling the creation of cross-chain oracles and other data-driven applications.
Challenges and Considerations
While cross-chain interoperability offers immense potential, it's important to be aware of the challenges and considerations involved:
- Security Risks: Cross-chain bridges can introduce new security risks, as they create a single point of failure that could be exploited by attackers.
- Complexity: Building cross-chain applications can be complex, requiring expertise in multiple blockchain platforms and protocols.
- Gas Costs: Transferring data and assets between chains can incur gas costs on both chains, which can impact the overall cost-effectiveness of cross-chain applications.
- Latency: Cross-chain communication can introduce latency, which can impact the performance of real-time applications.
Conclusion: The Future of Interconnected Blockchains
Cross-chain interoperability is a critical step towards a more interconnected and collaborative blockchain future. By connecting Solana and Ethereum with Wormhole, developers can unlock new possibilities for innovation and create applications that leverage the unique strengths of both platforms. As the blockchain space continues to evolve, cross-chain solutions like Wormhole will play an increasingly important role in driving adoption and unlocking the full potential of decentralized technology.
Frequently Asked Questions
1. What is cross-chain interoperability?
Cross-chain interoperability refers to the ability of different blockchain networks to communicate and interact with each other seamlessly. It enables the transfer of data and assets between chains, allowing for greater collaboration and innovation within the blockchain space.
2. Why is cross-chain interoperability important?
Cross-chain interoperability is important because it breaks down the silos between different blockchain ecosystems, allowing users and developers to access a wider range of services and opportunities. It also enables the creation of more complex and innovative applications that leverage the unique strengths of multiple chains.
3. What is Wormhole?
Wormhole is a generic cross-chain messaging protocol that enables communication between different blockchain networks. It acts as a decentralized bridge, allowing data and assets to be transferred securely and efficiently between supported chains.
4. How does Wormhole work?
Wormhole works by using a network of Guardians who observe and attest to events on connected chains. When a message is sent from one chain to another, the Guardians verify the message and generate a verifiable attestation, which is then relayed to the destination chain.
5. What are the benefits of using Wormhole to connect Solana and Ethereum?
Using Wormhole to connect Solana and Ethereum allows developers to build cross-chain applications that leverage the high performance of Solana and the established ecosystem of Ethereum. It also enables the seamless transfer of assets between the two chains, opening up new opportunities for DeFi, gaming, and other applications.